2-Navigating the RStudio Interface
- 1 Getting familiar with the RStudio Interface
- 2. Working in the Console
- 3. Working in the Code Editor
1 Getting familiar with the RStudio Interface
The RStudio interface is divided into several key areas, each serving a specific purpose.
- You can rearrange these windows and tabs to fit your personal preference by dragging them around the workspace. When you rearrange the panes in RStudio on your computer, the layout stays as you set it across future sessions.
1.1 Code Editor:
The code editor is where you write and edit your R scripts. It includes syntax highlighting, code completion, and other helpful tools to make coding easier.
1.2 Console:
The console is where R code is executed.
You can type commands directly into the console, like a scratch pad. It also displays outputs, messages, and errors.
You might prefer to use the console for immediate execution, or testing of small code snippets or commands.
1.3 Files/Plots/Packages/Help Pane:
Files: Browse, open, and manage files in your working directory.
Plots: View graphical outputs from your R code, such as plots and graphs.
Packages: Install, update, and load R packages.
Help: Access R documentation and help files for:
- Functions and packages
- Example code
- Information about datasets built in to R
- Information about other general R-related topics.
Viewer: Used to view local web content.
- We won’t be covering this
- E.g., web graphics generated using packages like - googleVis, - htmlwidgets - rCharts - local web application created using Shiny, Rook, or OpenCPU.
- See more at the RStudio Viewer Page
Presentation: You can use RStudio to create presentations (see more here.
1.4 Environment/History Pane:
Environment: Shows your current workspace, including:
- Defined variables
- Data frames
- Function objects.
History: Records all the commands you’ve run in the current and previous sessions.
Connections: Used to manage and configure the integration of data sources with your projects.
- E.g., Oracle, SQL, Salesforce
Tutorial: Used to run tutorials that will help you learn and master the R programming language.
- See more at the RStudio Tutorial Page
⭐ Task 1-1
Open RStudio
Get familiar with the interface by identifying the 4 windows and switching between the tabs. This task is just for you to get comfortable. There is no solution for this task.
2. Working in the Console
The console is the action center where your code gets executed immediately. It’s great for quick experiments or testing ideas, but it doesn’t save your work!
This is also where you install packages. Packages should be installed through the console, and loaded through the code editor.
Each new line of code (aka. command line) begins with the angle bracket >
also known as the ‘prompt’ symbol.
You will type the commands into the Console after the most recent angle bracket >
.
command line: lines of code in your console.
‘prompt’ symbol >
: Each new command starts with this.
execute: run your command by pressing the ‘enter’ or ‘return’ key on your keyboard.
- When you are ready to execute (‘run’) the command, type ‘enter’ or ‘return’ key on your keyboard.
- The output to the command will appear below your command.
Things to be mindful of:
-
You cannot execute a command until the previous command has been completely executed.
-
If you don’t see the prompt symbol, one of two things is happening:
-
R is still processing your previous command, and you must wait for it to finish.
-
You might instead see the plus
+
symbol, which indicates that you have entered an incomplete command. -
If you see the
+
symbol, you must enter the remainder of the command before entering a new one. -
An error will occur if you write the
+
symbol into your command. -
Sometimes the output can be extensive and show more information than you expected
-
E.g., when you load in a package (we will discuss packages more in Activity 3).
⭐ Task 2-1
Try getting help!
To do this, you’ll run the help()
function. - Try getting information on vectors.
Check Your Code
#Get additional information about "vectors" (a data type),
help("vector") # then type 'enter' or 'return'
help("vector")
will provide you with information about the mean function in RStudio. - The help information will be displayed in the Console following your command.
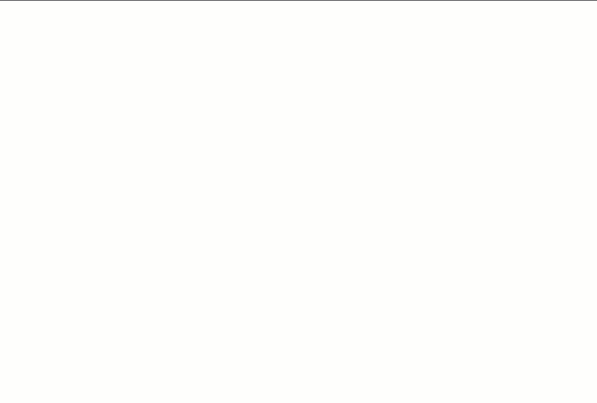
</details>
Note: You can get help on related content by selecting the dropdown list at the top of the Help tab.
3. Working in the Code Editor
The code editor is where you write and organize instructions in a script for R to follow. Think of it as a smart notepad that not only helps you learn and experiment but also allows you to save your work for later use. By writing code in the editor, you can easily reuse and adapt your scripts, and share them with collaborators
⭐ Task 3-1
Create a new R script.
- Click File > New File > R Script.
- Save your script: A blank script opens in the code editor. Save it by clicking
File
>Save
(choose a name and location).
- It is useful to store all related files in one folder, such as this script and any data you import/export here.
- A blank script opens in the code editor. Start typing your code as you would in the console.
3.1 Tips for writing code in an R script:
-
To add comments, begin your note with a
hash
# this is a comment
this is not a comment, and will cause an error
-
Use meaningful names (we will learn more about creating variables in the following activity page.)
# BAD naming a.dim1 <- 10 # height of object 1 b.dim2 <- 5 # length of object 2
# GOOD naming object1.length <- 10 object2.width <- 5
-
Order your code logically.
- If you want to run your code all at once (perhaps using a different data source), it will run each line in order.
3.2 Running your code
Run a single line of code:
-
Click anywhere on the line you want to run.
-
Press Ctrl + Enter (Windows/Linux) or Cmd + Enter (Mac).
Run multiple lines of code:
-
Highlight the lines you want to run.
-
Press Ctrl + Enter (Windows/Linux) or Cmd + Enter (Mac).
-
Say you have created two separate variables for different words, as well as a third variable that concatenates (combines) those two variables. You have also run all of the code line by line as you created each variable (Ctrl + Enter (Windows/Linux) or Cmd + Enter (Mac)).
rectangle1.length <- 10 # Line 1 rectangle1.width <- 5 # Line 2 # `*` is for multiplication rectangle1.area <- rectangle1.length * rectangle1.width # Line 3
-
You then you edit
rectangle1.length
to15
andrectangle1.width
to8
, but you don’t run each line as you edit it.rectangle1.length <- 15 # Line 1 rectangle1.width <- 8 # Line 2 rectangle1.area <- rectangle1.length * rectangle1.width # Line 3
- If you run only Line 1, the value of
rectangle1.length
will change, butrectangle1.width
andrectangle1.area
will not change. - If you run only Line 2, the value of
rectangle1.width
will change, butrectangle1.length
andrectangle1.area
will not change. - If you run only Line 3, no values will change.
- If you select lines 1, 2, and 3, all values will change.
- If you run only Line 1, the value of
Run the entire script:
-
Save the script (optional but recommended).
-
Click Source at the top-right corner of the script editor, or press Ctrl + Shift + Enter (Windows/Linux) or Cmd + Shift + Enter (Mac).
3.3 Deleting variables
Deleting the line of code that created a variable will not cause the variable to be removed.
To delete a variable, delete the line of code in the Code Editor, then rm()
function in the console.
# Line 1 is deleted
rectangle1.width <- 5 # Line 2
#`paste()` concatenates (combines) the values associated with each variable
rectangle1.area <- paste(rectangle1.length, rectangle1.width) # Line 3
> rm(rectangle1.length) # Run in the code editor to remove the variable from your entire environment
WARNING: If you remove a variable that another variable depends on, you will see the following error: Error: object 'varaible name' not found
.
Carefully consider the consequences of removing variables before doing so.
⭐ Task 3.3-1
Test editing variables.
Copy and paste the following code into your new R script (in the code editor).
-
Edit the values for
rectangle1.length
andrectangle1.width
, and run each line(s) of code in different orders to see what happens. -
Then try deleting different variables through the code editor and the console.
rectangle1.length <- 15 # Line 1
rectangle1.width <- 8 # Line 2
rectangle1.area <- rectangle1.length * rectangle1.width # Line 3
We’ll get to different types of variables in the next activity.
📍 As you work through these activities, remember to save your script(s) regularly.
- File
- Save (or cmd+s on Mac, ctrl+s on Windows)